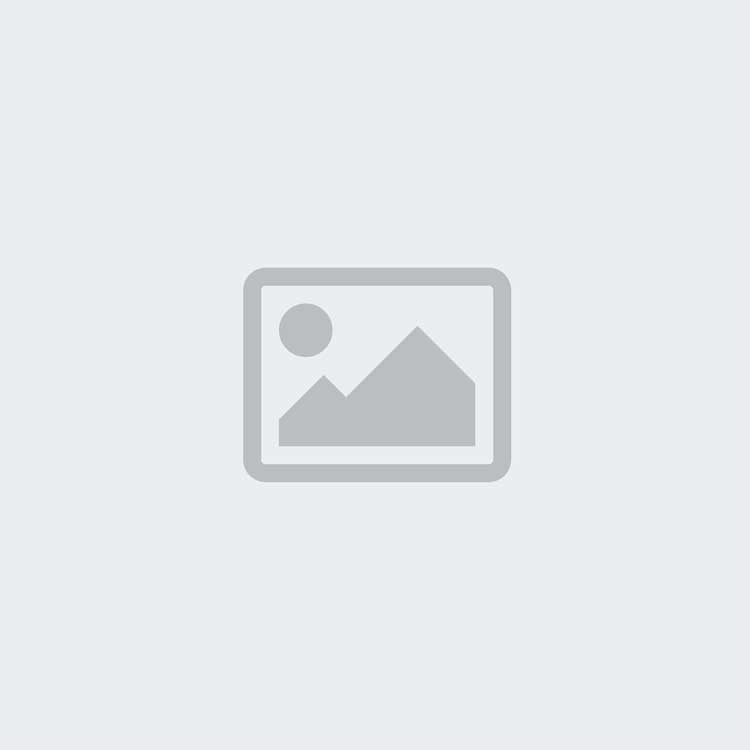
In computer programming, a constant is an identifier with an associated value which cannot be altered by the program during normal execution – the value is constant. This is contrasted with a variable, which is an identifier with a value that can be changed during normal execution – the value is variable. Constants are useful for both programmers and compilers: for programmers they are a form of self-documenting code and allow reasoning about correctness; while for compilers they allow compile-time and run-time checks that constancy assumptions are not violated, and allow or simplify some compiler optimizations. There are various specific realizations of the general notion of a constant, with subtle distinctions that are often overlooked. The most significant are: compile-time (statically-valued) constants, run-time (dynamically-valued) constants, immutable objects, and constant types (const). Typical examples of compile-time constants include mathematical constants, values from standards (here maximum transmission unit), or internal configuration values (here characters per line), such as these C examples: const float PI = 3.1415927; // maximal single float precision const unsigned int MTU = 1500; // Ethernet v2, RFC 894 const unsigned int COLUMNS = 80; Typical examples of run-time constants are values calculated based on inputs to a function, such as this C++ example: void f(std::string s) { const size_t l = s.length(); // … }